Gorelease
This post is about gorelease, and not goreleaser, which can cause some confusion especially when I was performing research on the former and kept getting results for the latter. Gorelease is a nifty tool in the golang.org/x/exp
module which analyzes the differences between the current code’s exposed API in your repository with the latest published code, and recommends a new semantic version to release. This is useful for Go library modules/packages which get imported by other Go code. Bumping your semantic versions properly is important to not break upstream codebases. For instance, no changes to the API layer would likely be a patch bump, new additions to your API might warrant minor bumps, while any and all breaking changes should be considered a major bump.
I recently quit my previous job and, while waiting for my next to begin, I was doing research on Gitlab pipelines. We had recently moved to Gitlab for our SCM and CI/CD but never got the chance to properly work with them or read their documentation. It proved insightful and I got a clear and better understanding of how they work and how I might’ve been able to use them effectively for my tasks, had I not quit.
One item that stood out to me was the multitude of ways in which Gitlab CI pipelines could run. Specifically, they’re not only run on new commits and tags but also for merge requests and new commits to merge requests. As well, a pipeline’s jobs can choose whether to run based on if its triggered for a merge request as opposed to just a regular commit (in addition to a variety of other factors) which can lead to pipelines that are more customized for an MR, for new commits, for deployments, and more.
This interested me, and I wondered how gorelease could be combined with Gitlab MR pipelines to automatically suggest a new version for an importable Go library module. This blog post is a simple retelling of my experimenting with these two concepts.
Gitlab Pipeline Research
A quick and simple explanation is that Gitlab pipelines are defined in a .gitlab-ci.yml
at the root of your code repository and define a set of jobs that execute to lint, build, test, deploy, and/or perform other actions against your code. The file defines a pipeline, which is made up of stages, each of which contain jobs to execute. Jobs are executed in isolate silos from each other, except if you use caching or artifacts to move results from one job to another explicitly.
build-job:
stage: build
script:
- echo "Hello, $GITLAB_USER_LOGIN!"
test-job1:
stage: test
script:
- echo "This job tests something"
test-job2:
stage: test
script:
- echo "This job tests something, but takes more time than test-job1."
- echo "After the echo commands complete, it runs the sleep command for 20 seconds"
- echo "which simulates a test that runs 20 seconds longer than test-job1"
- sleep 20
deploy-prod:
stage: deploy
script:
- echo "This job deploys something from the $CI_COMMIT_BRANCH branch."
environment: production
Jobs run in a scratch environment, which fetches the repository for code the referenced commit/tag as well as any job caches or previous job artifacts. Jobs by default run in an empty Docker container (this may be overly simplified), and you can customize which Docker image different jobs execute within. For instance, if you want a job to perform go build
you might use the golang:1.24-alpine
image, or for JS projects you could use a node image.
Go pipelines
I found a wonderful starting resource for quickly getting up and running with using Gitlab pipelines for Go projects: https://aarongoldenthal.com/posts/gitlab-ci-pipeline-for-go-projects/. This article and its linked repository containing importable pipeline code.
The implementation is opinionated and may not fit all use cases or preferences. For instance, it sets the GOPATH
to $CI_PROJECT_DIR/.go
which creates a .go
folder inside your repository which gets cached, but pollutes the clean working copy of the pulled repository code; this might force you to include .go
in your .gitignore
.
It also includes an software bill of materials (SBOM) job. This is geared towards shippable executables as opposed to importable library modules, and required some changes and overrides to get working. The author intends you to import this Gitlab pipeline confiuration from a URL, and Gitlab’s methods to override imported pipelines is not very clear or clean:
# Import
include:
- project: 'gitlab-ci-utils/gitlab-ci-templates'
ref: 'main'
file:
- '/collections/Go-Build-Test-Deploy.gitlab-ci.yml'
variables:
GO_BUILD_CURRENT: 'true'
GO_BUILD_MULTI: 'true'
GO_BUILD_NAME: 'testgopkg' # This was required to work for non-command modules as well
# Override the sbom job variables
go_sbom:
variables:
SBOM_TYPE: mod # Override sbom type to mod instead of app
SBOM_ARGS: "" # Remove sbom tool args
None of this was pertinent to toying with gorelease, but I thought it would be nice to have existing Go CI code to add to to make the experimenting a little bit more realistic.
One extra note is that services are very promising for setting up temporary PostgreSQL databases for code tests to run and not require external databases.
Gorelease
Once my pipeline was passing on regular builds, I could move on to making a merge request and trying out gorelease. I added the following job, which was copied and slightly modified from one of Aaron’s Go jobs.
go_release:
# Same image as the other jobs for quick image caching
image: golang:1.24.0-alpine3.21@sha256:2d40d4fc278dad38be0777d5e2a88a2c6dee51b0b29c97a764fc6c6a11ca893c
stage: mr
needs:
- job: go_mod_download # Only run after module cache is populated
optional: true
before_script:
# Install the gorelease tool
- go install golang.org/x/exp/cmd/gorelease@v0.0.0-20250218142911-aa4b98e5adaa
rules:
- if: $CI_PIPELINE_SOURCE == "merge_request_event" # Only run for MRs
script:
# Run gorelease
- gorelease
And quickly got my first error.
gorelease: could not find base version. Consider setting -version=v0.1.0 if this is a first release, or explicitly set -base=none: could not load versions for gitlab.com/test6464509/test: go: git init --bare in /go/pkg/mod/cache/vcs/d14f3c8570304a5fb154bf5a0dd17dfaad1dde713a0a7b610fe5f03af7b75fd8: exec: "git": executable file not found in $PATH
gorelease: could not find base version. Consider setting -version=v0.1.0 if this is a first release, or explicitly set -base=none: could not load versions for gitlab.com/test6464509/test: go: module gitlab.com/test6464509/test: git ls-remote -q origin in /Users/jamamp/go/pkg/mod/cache/vcs/d14f3c8570304a5fb154bf5a0dd17dfaad1dde713a0a7b610fe5f03af7b75fd8: exit status 128:
fatal: could not read Username for 'https://gitlab.com': terminal prompts disabled
Confirm the import path was entered correctly.
If this is a private repository, see https://golang.org/doc/faq#git_https for additional information.
Turns out the alpine-based golang images only have the Go sdk and no git executables installed. Go has git statically linked in its sdk, but that’s not available to gorelease
which apparently expects git to be installed locally to work. As well, when running this locally without my git properly setup I got authentication errors with Gitlab for the private testing repository. Gitlab CI jobs get their own authentication token which can be referenced and used to pull code and perform some other job-specific actions however, and I found a good snippet for easily fixing your job’s git without much hardcoded changes. Adjusting the pipeline a bit, we get:
go_release:
before_script:
# Add the git cli
- apk add git
# Automatically add the job's token to git config for gorelease to pull code.
# Note this is only needed since this repo is private and not available via proxy
- git config --global url.https://gitlab-ci-token:${CI_JOB_TOKEN}@${CI_SERVER_HOST}.insteadOf https://${CI_SERVER_HOST}
- export GOPRIVATE=${CI_SERVER_HOST}
- go install golang.org/x/exp/cmd/gorelease@v0.0.0-20250218142911-aa4b98e5adaa
gorelease: repo /builds/test6464509/test has uncommitted changes
Turns out gorelease doesn’t like when there are uncommitted git changes. This wasn’t clearly specified in its documentation, but it makes a lot of sense: uncommitted changes might not actually make it into the repo commits and thus may skew the results of a suggested semantic version bump. But this was running in a Gitlab pipeline and not my local working copy, what’s the deal? This was due to the various job artifacts and caches from the previous Go jobs that ran before this one: the Go module/package caches were in .go/
Running on runner-xs6vzpvo-project-67429682-concurrent-0 via runner-xs6vzpvo-s-l-s-amd64-1740428706-1a362f41...
Getting source from Git repository 00:02
Fetching changes with git depth set to 20...
Initialized empty Git repository in /builds/test6464509/test/.git/
Created fresh repository.
Checking out 45951d21 as detached HEAD (ref is main)...
Skipping Git submodules setup
$ git remote set-url origin "${CI_REPOSITORY_URL}"
Downloading artifacts 00:01
Downloading artifacts for go_mod_download (9230766223)...
Downloading artifacts from coordinator... ok host=storage.googleapis.com id=9230766223 responseStatus=200 OK token=...
Executing "step_script" stage of the job script
I could have adjusted my .gitignore
to mask out the .go
folder, but opted to simply stash and pop any git changes. It’s possible that other artifacts could be added to a pipeline and break this step again.
go_release:
script:
- git stash --include-untracked
- gorelease
- git stash pop
This ended up working well! The command suggested a new version in markdown format based on the changes between the latest published version and HEAD
. But, the results were hidden in the CI job logs. If this is running for a merge request, shouldn’t we see it in the MR more prominently? This would be greater UX so that suggest version changes are more visible to the engineers merging the MR and applying tags later.
Gitlab Merge Request Comments
After a small bit of digging, I found the Gitlab API responsible for posting comments (termed notes) to a merge request, and cobbled together a quick script to attempt to post this:
go_release:
before_script:
- apk add curl # Also needed to add curl which was not present in alpine
script:
- git stash --include-untracked
- gorelease | tee gorelease.txt # Gather the results into a file for the cURL post below
- git stash pop
- |
curl --request POST \
--header "JOB-TOKEN: ${CI_JOB_TOKEN}" \ # Use the job token for authentication
--header "Content-Type: application/json" \
--url-query body@gorelease.txt \ # Use the results of gorelease for the markdown body
# Use other env vars for all the required fields to work automatically for this project, repo, & MR
"${CI_API_V4_URL}/projects/${CI_PROJECT_ID}/merge_requests/${CI_MERGE_REQUEST_IID}/notes"
gorelease: repo /builds/test6464509/test has uncommitted changes
Facepalm! Creating a gorelease.txt
in the current working directory happens before/during the execution of gorelease, which interrupts gorelease from actually working. Adding gorelease.txt
to the .gitignore
cleared that up and I was then met with HTTP 401 Unauthorized.
I thought that using the Job’s temporary CI token would work well but according to the documentation, the tokens do not have Notes API access. There are some posts asking for this functionality, as it seems like it makes a lot of sense for an MR-driven Job to at least post a comment. But alas, it is not allowed. Instead, you must create an access token with better API privileges, configure that as a CI/CD variable in your project, and reference that for the API request.
When attempting to do so, I ran into a road block: project access tokens are not allowed on the free tier of Gitlab SaaS. I instead had to make a personal access token. This has the unfortunate side effect of making the MR comments appear as if I wrote them, though this could be remedied by making a “bot” account and making PATs from it. As well, when creating either project or personal access tokens, Gitlab infamously doesn’t allow for more granular API permissions. You must create a token with either full API read or read/write access, and can not limit it to just the Notes API for instance.
The combination of all of this would make it difficult to create or justify a generic tool for publishing a gorelease-based Gitlab CI job for others to use. You have to ask the implementer to create a token, configure it as a variable which other jobs could potentially reference, which has too much API access, and requires paid tier access or bot accounts to be cleaner.
Eventually it did begin working, albeit as a somewhat complicated proof of concept:
go_release:
image: golang:1.24.0-alpine3.21@sha256:2d40d4fc278dad38be0777d5e2a88a2c6dee51b0b29c97a764fc6c6a11ca893c
stage: mr
needs:
- job: go_mod_download
optional: true
before_script:
- apk add git
- apk add curl
- git config --global url.https://gitlab-ci-token:${CI_JOB_TOKEN}@${CI_SERVER_HOST}.insteadOf https://${CI_SERVER_HOST}
- export GOPRIVATE=${CI_SERVER_HOST}
- go install golang.org/x/exp/cmd/gorelease@v0.0.0-20250218142911-aa4b98e5adaa
rules:
- if: $CI_PIPELINE_SOURCE == "merge_request_event"
script:
- env
- git stash --include-untracked
- gorelease | tee gorelease.txt
- git stash pop
- |
curl --request POST \
--header "PRIVATE-TOKEN: ${GL_CI_API_TOKEN}" \
--header "Content-Type: application/json" \
--url-query body@gorelease.txt \
"${CI_API_V4_URL}/projects/${CI_PROJECT_ID}/merge_requests/${CI_MERGE_REQUEST_IID}/notes"
$ curl --request POST \ # collapsed multi-line command
% Total % Received % Xferd Average Speed Time Time Time Current
Dload Upload Total Spent Left Speed
100 852 100 852 0 0 1661 0 --:--:-- --:--:-- --:--:-- 1664
{"id":2364987940,"type":null,"body":"# gitlab.com/test6464509/test\n## incompatible changes\nVersion: value changed from \"0.0.1\" to \"v0.1.0\"\n## compatible changes\nId: added\n\n# summary\nInferred base version: v0.1.0\nSuggested version: v0.2.0","attachment":null,"author":{"id":26355958,"username":"jamamp","name":"James Linnell","state":"active","locked":false,"avatar_url":"https://secure.gravatar.com/avatar/0c5344723fa8ff395f17f42a4630d9f94568d122c1e4ae37f0f2aa3527e51325?s=80\u0026d=identicon","web_url":"https://gitlab.com/jamamp"},"created_at":"2025-02-25T02:59:30.494Z","updated_at":"2025-02-25T02:59:30.494Z","system":false,"noteable_id":364584221,"noteable_type":"MergeRequest","project_id":67429682,"resolvable":false,"confidential":false,"internal":false,"imported":false,"imported_from":"none","noteable_iid":1,"commands_changes":{}}
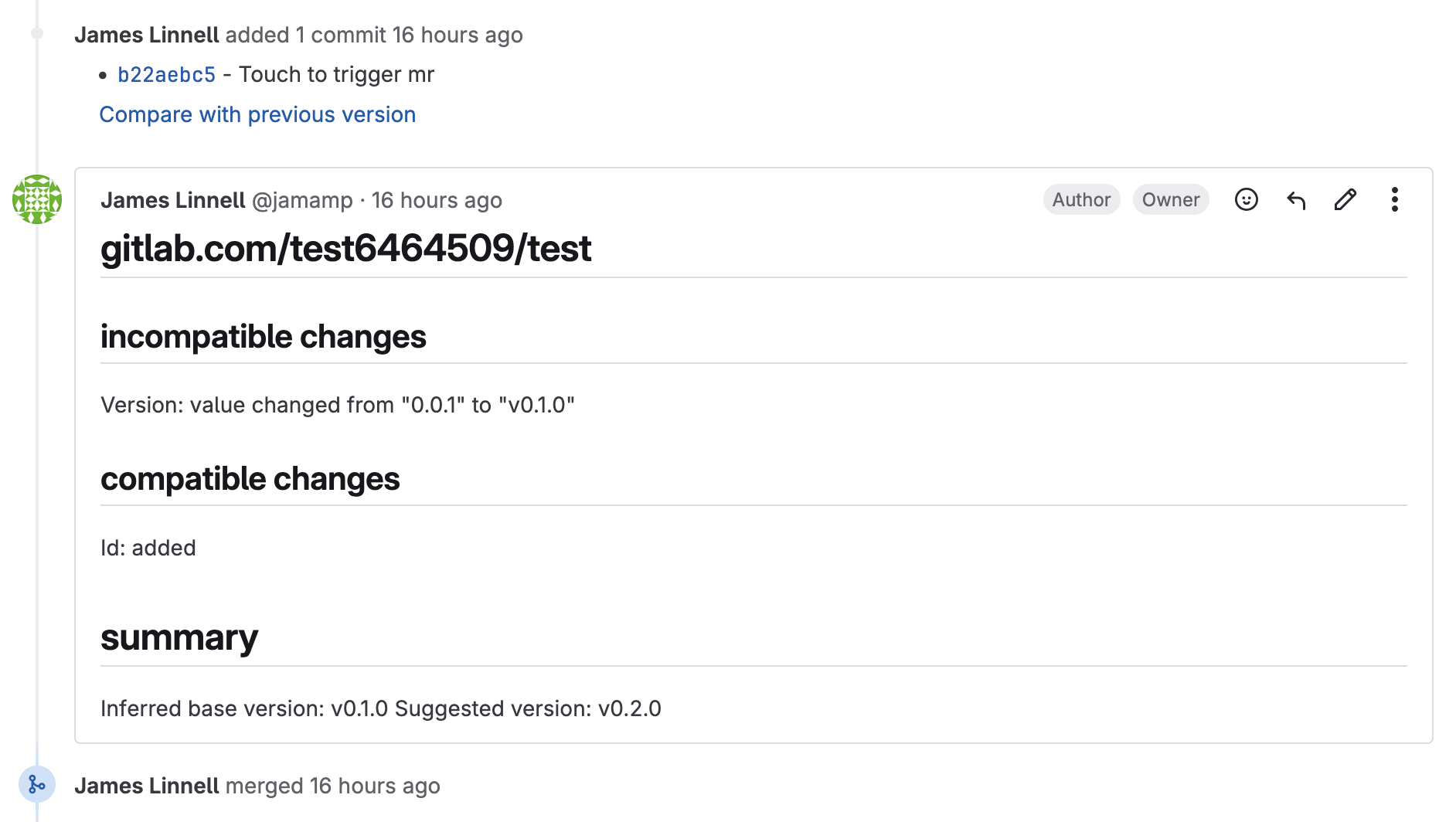
Posted comment on a merge request from a CI gorelease job
I was able to close this sample merge request and tag the resulting merge commit with an appropriately suggested version bump. Gorelease is also nice enough to explain the reasoning behind its suggestion: it lists our incompatible and compatible changes to the API layer. My test was rather simple with only exposing a couple of string constants, but in practice it should be capable of much more complicate changes.
Closing
One interesting item to note, that I haven’t tested much yet, is that the gorelease
program will failed with a non-zero exit code when there exist breaking changes in the Go module’s exported API and without an accompanying /v2
suffix to the module path. This would result in a CI job failure, which could prevent the merging of the merge request. And for good reason!
While gorelease is still in in the experimental module, it lends itself well to pipeline automation and other workflows. Aside from the wart of uncommitted git changes, it has a variety of use cases and outputs nice markdown which is compatible with MR comments, and stops CI when incompatible changes aren’t matched with an explicit /v2
bump. It’s not critical to the functioning of a well-oiled Go CI pipeline, but helps automated the boring work of semver bumping and can prevent confusion among teammates not used to performign these kind of changes.
This might be difficult to combine with other automatic tools that handle semver bumping for you. For instance, many workflows require developers to appropriately label their git commits with conventional commits and automatically infer patch/minor/major version bumps from those notes, as well as automatically create release notes from the commit messages themselves. Gorelease only looks at the exposed API layer of Go packages, and is only a suggestion which may not be easy to action upon automatically. It would fail to discover breakings changes outside of the API; that is, change in functionality of exposed functions. But, I believe it could be a good safety catch, possibly when combined with manually-driven conventional commits.
Gitlab Pipeline Takeaways
Gitlab pipelines are slow! I didn’t tinker with any of the Gitlab CI runner details, and only used all of the default settings. Due to running individually on different docker images, the jobs took a while to start up and prepare themselves before actually executing the few steps per job. Gitlab states an entire pipeline took 1m30s to execute. During execution, I did notice that full parallelism was not achieved; perhaps Gitlab limits parallel jobs in the free tier to save on resources and some jobs get slightly delayed (this is totally fine and expected, I would do the same).
But, doing all of this in a Dockerfile or otherwise a single job, I feel, would be so much faster. The downside is your only have one base image and may need to install several tools into it for functionality, such as adding git, curl, installing the gorelease and sbom go tools, and potentially more. Separating out jobs to run individually from each other keeps the tasks well isolated and clean, but comes at a loss in efficiency. This can lead to some DX frustration as you have to wait for complicated pipelines to complete.
Not only are Gitlab pipelines slow to execute, but the pipeline interface is also slow to update! My previous experience was using Jenkins jobs which provide a nice, quick, and live output in the console log. Gitlab on the other hand seems to only update every 10 seconds or so, which can be frustrating to watch. There’s no refresh button so you have to refresh the whole page if you want a more up to date representation of the pipeline or job state. Setup a websocket and listen for events more quickly!
The wart of the CI_JOB_TOKEN
not being able to add comments to a merge request is also frustrating. I feel like that could be a quick and simple win and greatly simplify some automated processes. That said, the existence of a job token that’s always there for you automatically for other tasks is super useful and I appreciate that a lot.
Github
I assume Github Actions can achieve very similar results. That would be another fun experiment to run: get gorelease to add suggestions as MR comments. Perhaps they have better DX with regard to API authorization and the like.